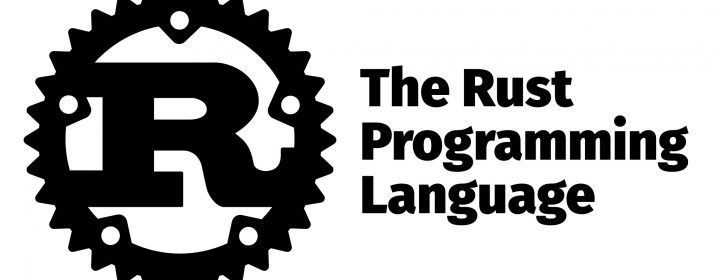
Getting Started with Rust
Learn the basics of Rust
Rust is a systems programming language that focuses on safety, concurrency, and performance. Developed by the Mozilla Foundation, Rust is designed to be a safer and more reliable alternative to languages like C and C++.
One of the key features of Rust is its emphasis on memory safety. Unlike languages like C and C++, which allow programmers to manipulate memory directly, Rust uses a compile-time borrow checker to prevent common memory-related bugs, such as null or dangling pointer references. This means that Rust programs are less likely to crash or have undefined behavior.
Rust also has strong support for concurrent programming, allowing multiple threads to run in parallel without the need for locks or other synchronization mechanisms. This makes it easier to write efficient and scalable programs that can take advantage of modern hardware.
In addition to its safety and concurrency features, Rust also has a powerful type system and pattern matching facilities that make it easy to write concise and expressive code. For example, Rust has algebraic data types, which allow you to define complex data structures using a combination of primitive and composite types. You can then use pattern matching to decompose and manipulate these data structures in a safe and efficient way.
Overall, Rust is a versatile and powerful language that is well-suited for a wide range of applications, from low-level systems programming to high-level
Install Rust
The first step to getting started with Rust is to install the Rust compiler and tools on your computer. You can do this by following the instructions on the Rust website: https://www.rust-lang.org/tools/install.
Write and run your first Rust program
Once you have Rust installed, you can write and run your first Rust program. To do this, create a new file called hello.rs
and add the following code:
fn main() {
println!("Hello, world!");
}
To run this program, open a terminal and navigate to the directory where you saved hello.rs
. Then, run the following command:
rustc hello.rs
This will compile the program and create an executable file called hello
. You can then run the program by typing ./hello
in the terminal. You should see the output “Hello, world!” printed to the screen.
Explore the Rust community and resources
To continue learning Rust, you can explore the many resources and community forums available online. Here are some good places to start:
- The Rust website (https://www.rust-lang.org) has a wealth of information, including documentation, tutorials, and examples of Rust code.
- The Rust forum (https://users.rust-lang.org) is a great place to ask questions, share your code, and connect with other Rust developers.
- The Rust documentation (https://doc.rust-lang.org) is a comprehensive reference for the Rust language and its standard library.
I hope this tutorial helps you get started with Rust! Happy coding!